基礎設定
canvas是原生的 html 標籤,可以用js來操控,繪製各種動畫或圖案。以下介紹基礎設定
1
| <canvas id="canvas"></canvas>
|
1 2 3 4 5 6 7 8
| const canvas = document.querySelector('#canvas');
const ctx = canvas.getContext('2d');
canvas.height = window.innerHeight; canvas.width = window.innerWidth;
|
繪製線條
基礎設定好後,可以嘗試來繪製線條。
- moveTo(x, y): 設定起始點的座標
- lineTo(x, y): 下一個點的座標
- stroke(): 依據座標繪製線條
1 2 3 4 5 6 7
| ctx.beginPath();
ctx.moveTo(50, 50);
ctx.lineTo(100, 50); ctx.stroke();
|
繪製後會出現水平的線條
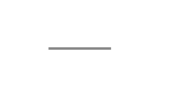
三角形
三角形一樣用lineTo指定點的座標,連回原點即可。
1 2 3 4 5 6
| ctx.beginPath(); ctx.moveTo(50, 50); ctx.lineTo(100, 50); ctx.lineTo(50, 100); ctx.lineTo(50, 50); ctx.stroke();
|
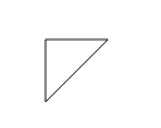
或者在最後用 closePath()可以自動連回原點。
1 2 3 4 5 6
| ctx.beginPath(); ctx.moveTo(50, 50); ctx.lineTo(100, 50); ctx.lineTo(50, 100); ctx.closePath(); ctx.stroke();
|
設定顏色與線條寬度
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| ctx.lineWidth = 5;
ctx.strokeStyle = '#000';
ctx.fillStyle = 'red'; ctx.beginPath(); ctx.moveTo(50, 50); ctx.lineTo(100, 50); ctx.lineTo(50, 100); ctx.closePath(); ctx.stroke();
ctx.fill();
|
經過設定顏色後的三角形
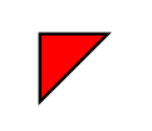
正方形
正方形一樣可用lineTo指定座標的方式繪製,但canvas有內建繪製正方形的函式。
1
| ctx.rect(x,y, w, h) // x,y一樣是座標,w和h是四邊形的寬和高*/
|
1 2 3 4 5 6
| ctx.lineWidth = 5; ctx.strokeStyle = '#000'; ctx.fillStyle = 'red'; ctx.rect(50, 50, 100, 100); ctx.stroke(); ctx.fill();
|
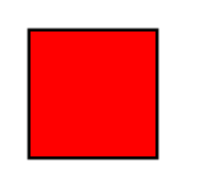
圓形
圓形的繪製使用 arc() 函式 來完成
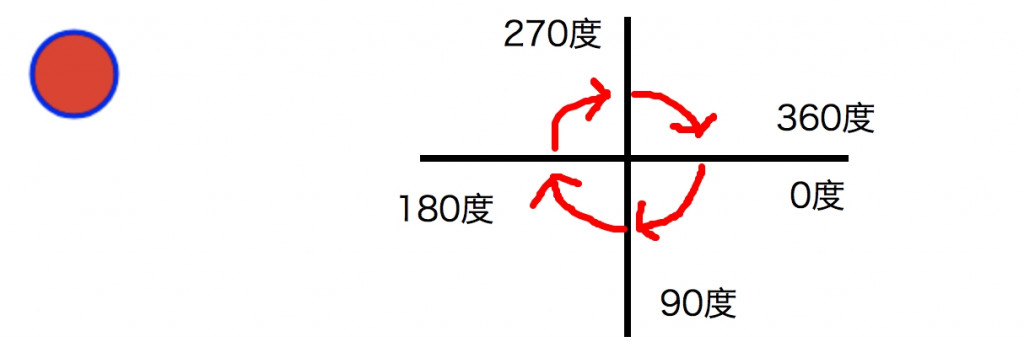
1 2 3 4 5 6
| ctx.lineWidth = 5; ctx.strokeStyle = '#000'; ctx.fillStyle = 'red'; ctx.arc(100, 100, 25, 0, Math.PI * 2); ctx.stroke(); ctx.fill();
|
因為 Math.PI 代表 180度,所以從 0度 到 Math.PI * 2 就可以畫出 360度的圓形了。
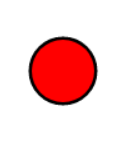
若要繪製半圓則改變起始的角度即可
1 2 3 4 5 6
| ctx.lineWidth = 5; ctx.strokeStyle = '#000'; ctx.fillStyle = 'red'; ctx.arc(100, 100, 25, Math.PI / 2, Math.PI * 1.5); ctx.stroke(); ctx.fill();
|