建立專案環境
先建立 index.html、all.js、all.css
1 2 3
| - index.html - all.js - all.css
|
在 index.html 中引入 gasp cdn
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <!DOCTYPE html> <html lang="zh-Hant-TW"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Document</title> <link rel="stylesheet" href="all.css" /> </head> <body> <div class="blueBox"></div> <script src="https://cdn.jsdelivr.net/npm/gsap@3.12.5/dist/gsap.min.js"></script> <script src="all.js"></script> </body> </html>
|
在 all.js 即可寫入 gasp 基本語法
1 2 3 4 5 6 7 8 9
| const blueBox = document.querySelector(".blueBox");
gsap.to(blueBox, { x: 500, duration: 3, rotation: 180, backgroundColor: "red", ease: "bounce.in", });
|
to、from、fromTo 語法
- to: 預設位置 => 指定位置
- from: 指定位置 => 預設位置
- fromTo: 同時設定起點和終點
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| const blueBox = document.querySelector(".blueBox");
gsap.to(blueBox, { x: 500, duration: 3, rotation: 180, backgroundColor: "red", ease: "bounce.in", });
gsap.from(blueBox, { x: 500, duration: 3, rotation: 180, backgroundColor: "red", ease: "bounce.in", });
gsap.fromTo( blueBox, { opacity: 0, duration: 2, rotation: 180, backgroundColor: "red", }, { opacity: 300, duration: 2, rotation: 180, backgroundColor: "pink", } );
|
delay
若希望動畫能在指定時間後再撥放,可用 delay 語法
1 2 3 4 5 6 7
| gsap.to(greenBox, { x: 500, duration: 5, backgroundColor: "blue", delay: 1.5, });
|
repeat
可使用 repeat 指定動畫重複次數
1 2 3 4 5 6 7 8
| gsap.to(greenBox, { x: 500, duration: 5, backgroundColor: "blue", delay: 1.5, repeat: 2, });
|
repeat 設定 -1 可以不斷執行動畫
1 2 3 4 5 6 7
| gsap.to(greenBox, { x: 500, duration: 5, backgroundColor: "blue", repeat: -1, });
|
動畫速率設定
動畫速率可以參考 官網 來設定。
1 2 3 4 5 6
| gsap.to(target, { duration: 2.5, ease: "power4.out", y: -250, });
|
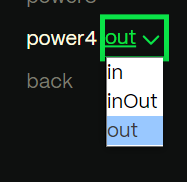
每種速率都可選擇 out in 等模式,可以在官網查看效果。
timeline 時間軸群組化
如果有多個物件要設定彼此動畫先後順序,可以用 timeline 群組化,就不用每一個用 delay 自己算延遲時間。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| const blueBox = document.querySelector(".blueBox"); const greenBox = document.querySelector(".greenBox");
const timeline = gsap.timeline();
timeline.to(blueBox, { x: 300, duration: 2, backgroundColor: "red", });
timeline.to(greenBox, { x: 500, duration: 5, backgroundColor: "blue", });
|
如果有兩個物件想等第一個物件動畫完後再一起跑時,可以設定 -= 語法。
blueBox、 greenBox 會在 pinkBox 跑到第二秒時一起跑。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| const timeline = gsap.timeline();
timeline.to(pinkBox, { x: 500, duration: 5, backgroundColor: "blue", });
timeline.to( blueBox, { x: 500, duration: 3, backgroundColor: "red", }, "-=3" );
timeline.to( greenBox, { x: 500, duration: 3, backgroundColor: "blue", }, "-=3" );
|